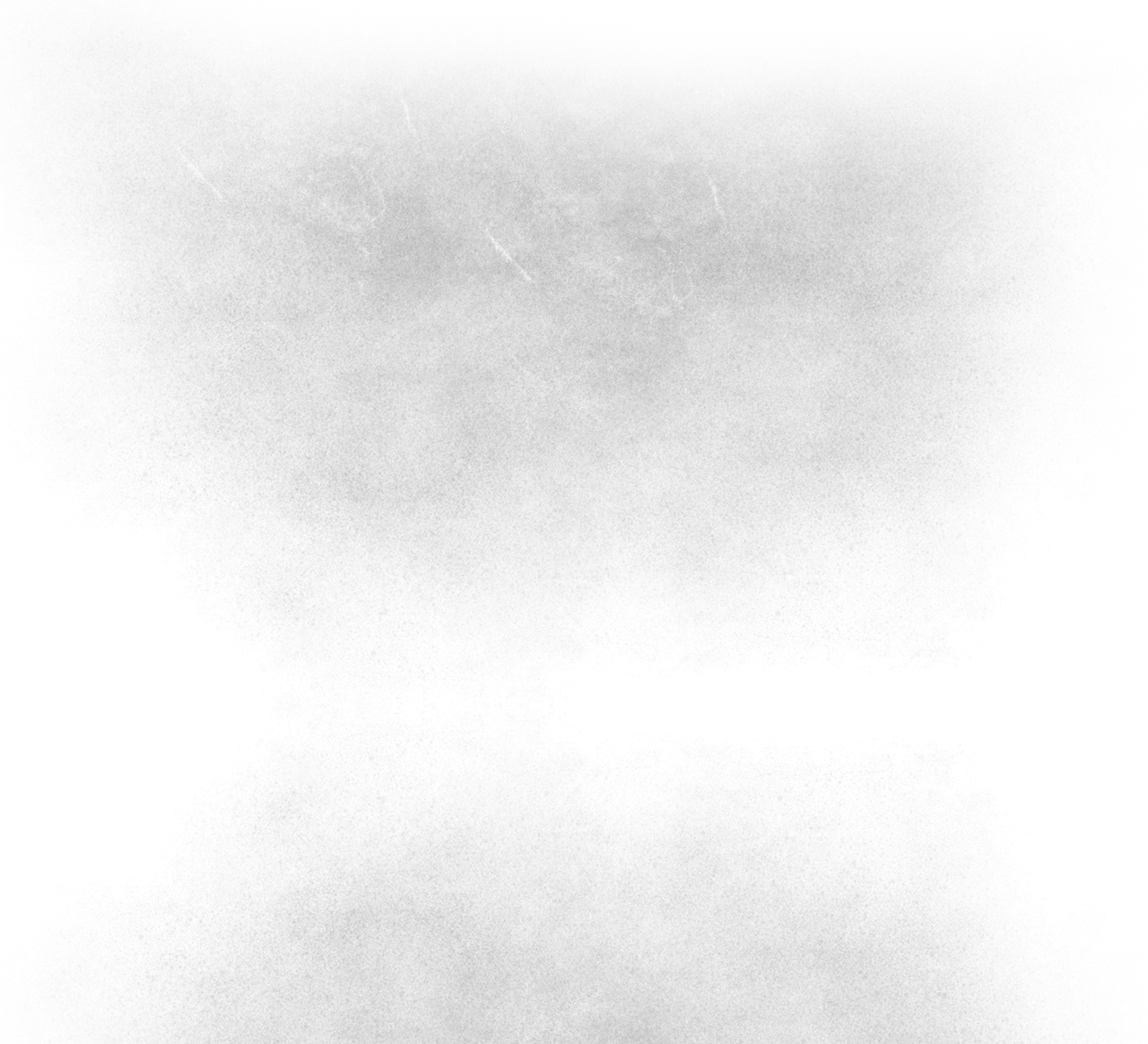

​Turbo C​

Conditionals, Loops, Arrays
There are a few control structures in C that you can make use of, very similar in syntax to those in Perl and PHP.

If/Else
These allow you to execute code IF a boolean expression is true:

if(1 == 1) {
printf("1 is equal to 1");
}
else {
printf("1 is not equal to 1");
}

 This speaks for itself really. If 1 is equal to 1, print out so, if not, print out that it isn't. Obviously 1 will always be equal to 1, so the printf() statement between the curly braces after the if() function will be executed.

If 1 wasn't equal to 1, then the else would be executed.

#include <stdio.h>
int main() {
int number;
printf("Enter a number:");
scanf("%d", &number);
if(number == 1) {
printf("The number you entered is 1.");
}
else if(number == 2) {
printf("The number you entered is 2.");
}
else if(number == 3) {
printf("The number you entered is 3.");
}
else if(number == 4) {
printf("The number you entered is 4.");
}
else {
printf("The number you entered is not 1, 2, 3 or 4.");
}
}

 It's pretty easy to see what's going on here, these functions allow is to test if a boolen expression, or series of boolean expressions are true, and execute code depending on those tests.
Boolen Expressions

 a == b /* is a equal to b */
a != b /* is a not equal to b */
a /* is a true */
!b /* is a not true */
a > b /* is a greater than b */
a < b /* is a less than b */
a = > b /* is a greater than or equal to b */
a = < b /* is a less than or equal to b */
a && b /* a AND b */
a || b /* a OR b */

 Any expression that returns TRUE will cause a conditional statement to execute the code contained within its brackets. The computer sees a boolean expression as either true or false. 0 is false, and anything else is true.
While

The while function is a loop, while the expression is true it will continue to execute the code in its brackets.

int a = 0;
while(a < 10) {
print("%dn", a);
a++; /* add one to a */
}

 This simply means, while the expression is true, execute the code between the curly brackets. The while function checks the expression, if it's true it executes the code then jumps back and tests the expression again, looping until it checks and finds the expression is false, then it continues executing any code after the while() function. In the example above it keeps checking and adding one to a each time, when a reaches 10, it is no longer less than 10, as stated in the expression, so it will discontinue its loop. So the example will print out the numbers 0 to 9.
​
We can also do:

 int a = 0;
do (
print("%dn", a);
a++; /* add one to a */
}
while(a < 10);

 Which is the same thing, do the code between the curly braces while the expression in the while() statement is true. If the statement is never true, the code in the braces will not be executed at all. Remember when checking for equality in a boolean expression, don't confuse a = b with a == b. = is the operator we use to assign a value to a variable, so if we use a single = it will assign b to a and then check whether a is true or not (effectively checking if b is true).
​
For

 We could do the same as we did in the while() examples with for(). For() allows us to include everything in one line.

for(a = 0; a < 10; a++) {
print("%dn", a);
}

 This is neater, it allows us to initialise the variable with a value, then test the expression, then perform an action on the variable. The code will execute while the expression is true, and if it is true, it performs the action and then executes the code between the braces. So we initialise a at zero, test if it's les than 10, if it is increment it, print out the value in a, and then go back and check the expression again, until it's no longer true. For() allows more:

 for(a = 0, b = 0; a < 10 && b < 20; a++, b = b + 2) {
print("a is %d and b is %dn", a, b);
}

 Seperating them with commas, we can include multiple initialisation variables and perform multiple actions, we cannot use a comma in the boolen expression, although we can test multiple conditions by using the AND (&&) sign. This will initialise both variables at zero, check to see whether a is less than 10 AND b is less than 20, while this expression is true execute. When the expression is false discontinue the loop, effectively counting from 0 - 9 with a, and counting up in two's with b.
Remember, although you initialise the variables with a value in for(), you still have to declare them first. Have a play around with these functions, get used to them, and when you're ready read on...
Arrays

 In this chapter we're going to use a new variable arrangement called an array. An array lets you declare and work with many variables of the same type. Say we wanted four integers:

int a, b, c, d;

Pretty easy, but what if we wanted 500? An easier way to do this is:

int a[4];
This is an array. The four integers inside the array are accessed with an index, it works like so:

 a[0]; /* first integer in array */
a[1]; /* first integer in array */
a[2]; /* first integer in array */
a[3]; /* first integer in array */

 Remember, although we define the array with four items, the index starts at 0. So the last item in the array is accessed with an index value one lower than the number of items in the array.
​
We can loop through the items in an array, the following code initialises all the values in the array as 1:

 int a[6], i;
for(i = 0; i < 6; i++) {
a[i] = 1;
}

 Remember the for() loop from the last chapter? It loops through while i is less than 6, incrementing i each time and assigning 1 to each item in the array, i represents the incrementing array index. C has no range checking, so if you index past the end of an array you will be manipulating memory beyond the range of that array, effectively overwriting data in memory that you shouldn't be. This will cause a crash or garbled data.

Compared to a normal variable.
Array "a" index
1 a[0]
1 a[1]
1 a[2]
1 a[3]
1 a[4]
1 a[5]

variable "b" index
1 b
You would change the value in the array with something like a[3] = 5; yet for the variable, simply b = 5;. An array takes up consecutive addresses in memory, it is an array of variables.

You will see more of arrays as you progress, it's hard to understand many concepts until you see the advantages when you actually use them.