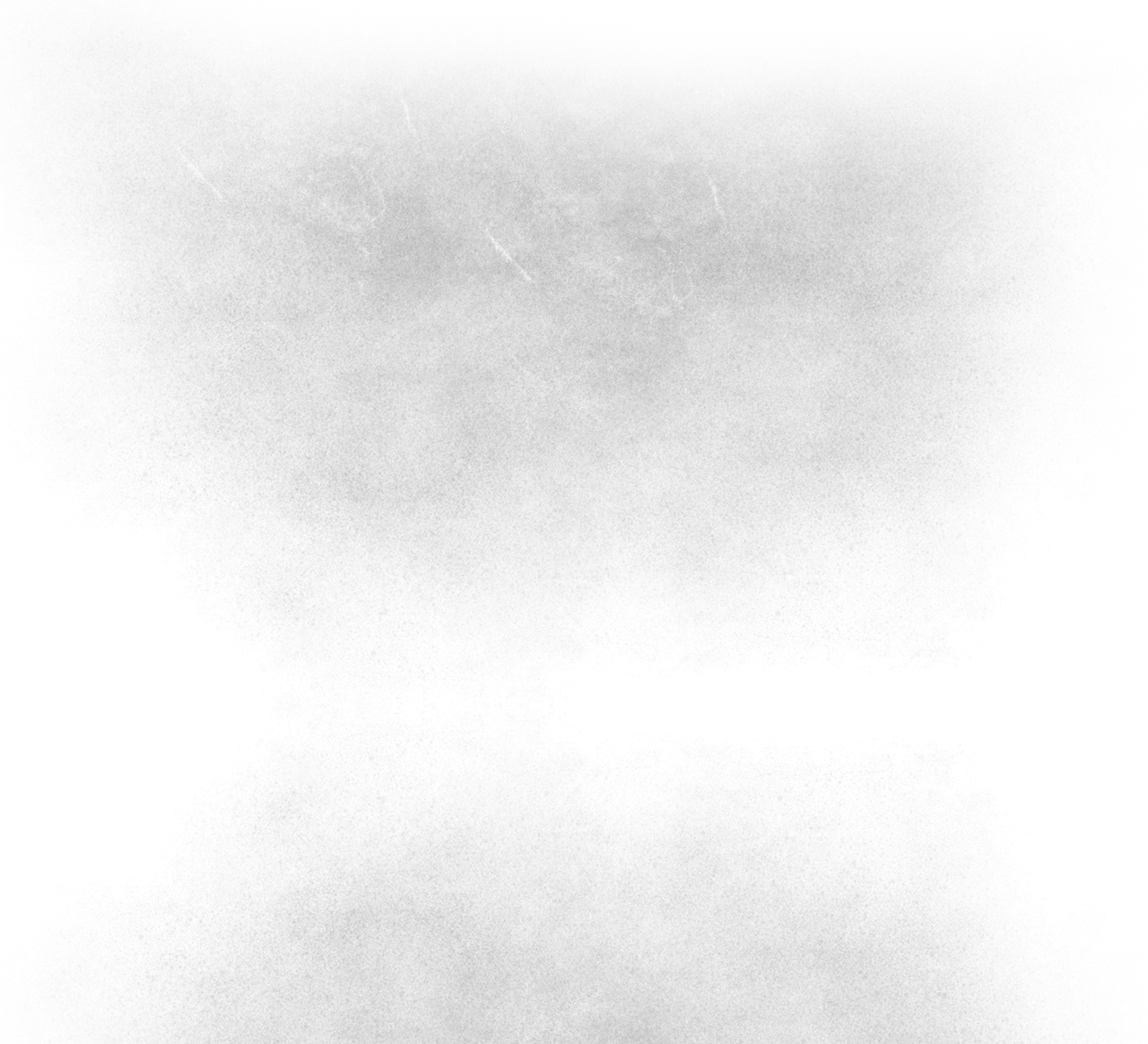

​Turbo C​

Defining variables in C is pretty simple. Again, if you know Perl or PHP this may seem a little strange, alot of C might, but it's worth learning this powerful language.
​
When we define a variable in C we must define what type it is, aswell as its name.
"The number is %d"

 What's that %d? That's where the value stored in the variable will be printed out. The second parameter is the variable name, and the %d is the place in the printf() string where we want that value to appear. If we did:
​int FirstNumber, SecondNumber;
​
We could define as many variables as we wanted. When we make a variable we are giving a name to our data. Any data you store in that variable is stored in a location in memory associated with that variable. So when we use the variable in later functions or operations, you can access it by a name, it makes it easier for us humans to solve problems this way.
​
We can also define a variable and give it a value all in one go:

int FirstNumber = 5;
More on printf()

Let's have a look deeper into printf(), let's use a variable with it.

 #include <stdio.h>
int main()
{
int Number = 4;
printf("The number is %d", Number);
}

 We have used an extra parameter with printf(). If we want to use a variable with printf() we must first enter the text to be printed, follow it by a comma, and then the variable name.
​int MyNumber;
​
The above define a variable named "MyNumber" that can handle integer values. Meaning this variable can only contain number values, not fractional numbers, whole numbers. There are a few types of variables:

 int MyNumber; /*int variable.*/
char MyLetter; /*char, can contain letters.*/
float MyFraction; /*float, can contain fraction numbers.*/
double MyExponential; /*can contain exponential values*/

 We must know what kind of data the variable will contain, variable names are case-sensitive, We cannot have two variables with the same name, we cannot have two functions with the same name. We can also define two variables of the same type in one go:
printf("The number %d is", Number);

 The program would read "The number 4 is", get it? As there are many types of variable, we must use the correct sign to output a variable in printf(), there are different ones depending on the type of data contained in the variable.

 %d /*integer input*/
%c /*chatacter input*/
%s /*string input*/
%f /*fractional input*/

So if the variable contained a character:

 #include <stdio.h>
int main()
{
char Letter = 'S';
printf("The letter is %c", Letter);
}

 We would use %c to show that the data stored in "Letter" is the type "char". We can use many variables in a printf() function.

 #include <stdio.h>
int main()
{
int Number = 3, Number2 = 5, Sum = Number + Number2;
printf("The sum of %d and %d is %d", Number, Number2, Sum);
}

 Will print "The sum of 3 and 5 is 8". Just keep the %d signs in order with the variable parameters on the right, as simple as that.
Variables