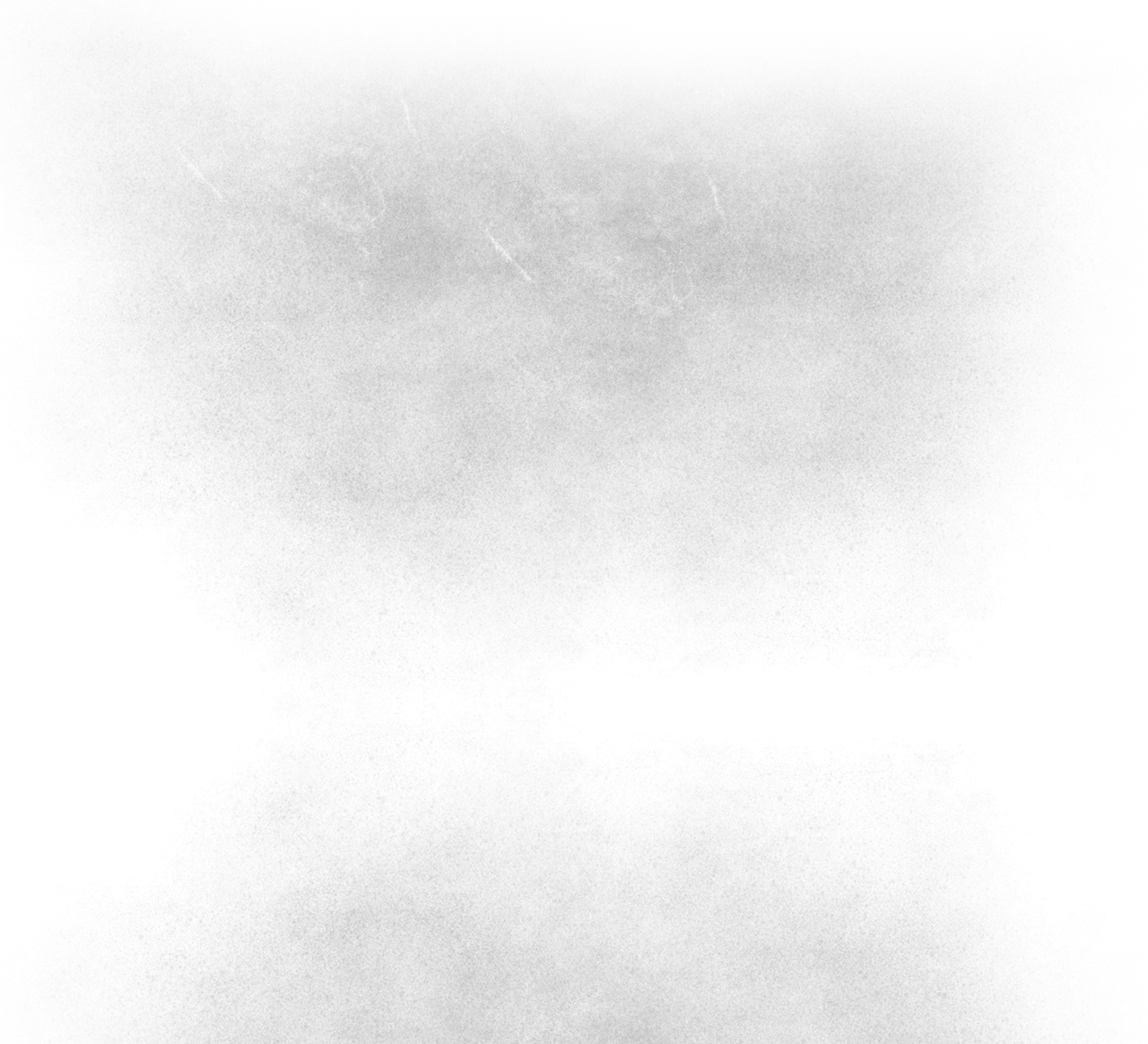

​Turbo C​
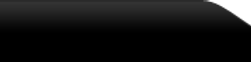
Sample Programs
HELLO WORLD
​
Take a look at the simple program below:
​
#include <stdio.h>
int main()
{
/*My first program*/
printf("Hello World!n");
return 0;
}
​
If you're completely new to C that'll probably look confusing, so let's go through it:
#include <stdio.h>

This line does what it says it does, it includes the header file "stdio.h" - stdio means "standard input/output. This "header" file contains ready-to-go functions for your program. Without this file you would have to write your own functions to input and output data, but why should you when others have done it for you? You can simply include their functions that are stored in the header file into your program and use these functions. If you know Perl or PHP this may seem weird (it did for me) but C's functions aren't "built-in" as they are in Perl and PHP, you have to include the appropriate header files containing the functions you wish to use in your program.
​/*My first program*/
This line is a comment, anything between /* and */ is a comment and will not be compiled. Comments are used to, of all things, comment your source code. If you get into C and start writing programs that interest others, sooner or later others will be reading your source code. To help them follow it more easily comment it, tell them what's happening at each step, commenting = good. A comment can span multiple lines, just contain it between the comment symbols.
​int main()
​
We have included our header file and have the functions contained within it available to us, now we need to make the program do something. But before we do anything we must tell the program where to start and where to finish.

int main()
{
/*program instructions*/
}

This defines where the program starts and where it ends, we contain the program instructions between the curly braces. All C programs must contain this if nothing else.
​printf("Hello World!n");
​
This is the printf() function. It is contained within the "stdio.h" file we included earlier. Our little program simply prints "Hello World!" to the screen. This is what prinf() does. We don't need to cover functions yet, just take note of this, we give printf() the string "Hello World!" and printf() outputs it (prints it to the screen). printf() has more features, we'll see one of these in the next chapter. The n character just means a new line, so after we print "Hello World!" take the pointer down to the next line.
​return 0;
​
This tells the main() function to return, in this case it just means end it. This isn't necessary, it depends which compiler you're using, so it's a good idea to include it just incase.
​
Save this little program to a file called "hello_world.c". You need a compiler (see last chapter). Then compile it and watch what it does, congratulations you just compiled your first C program.
​
This is the extreme basics of C, but we all have to start somewhere, the hardest part if you're completely new to programming is getting started, hopefully this chapter got you started and explained a few things to you.