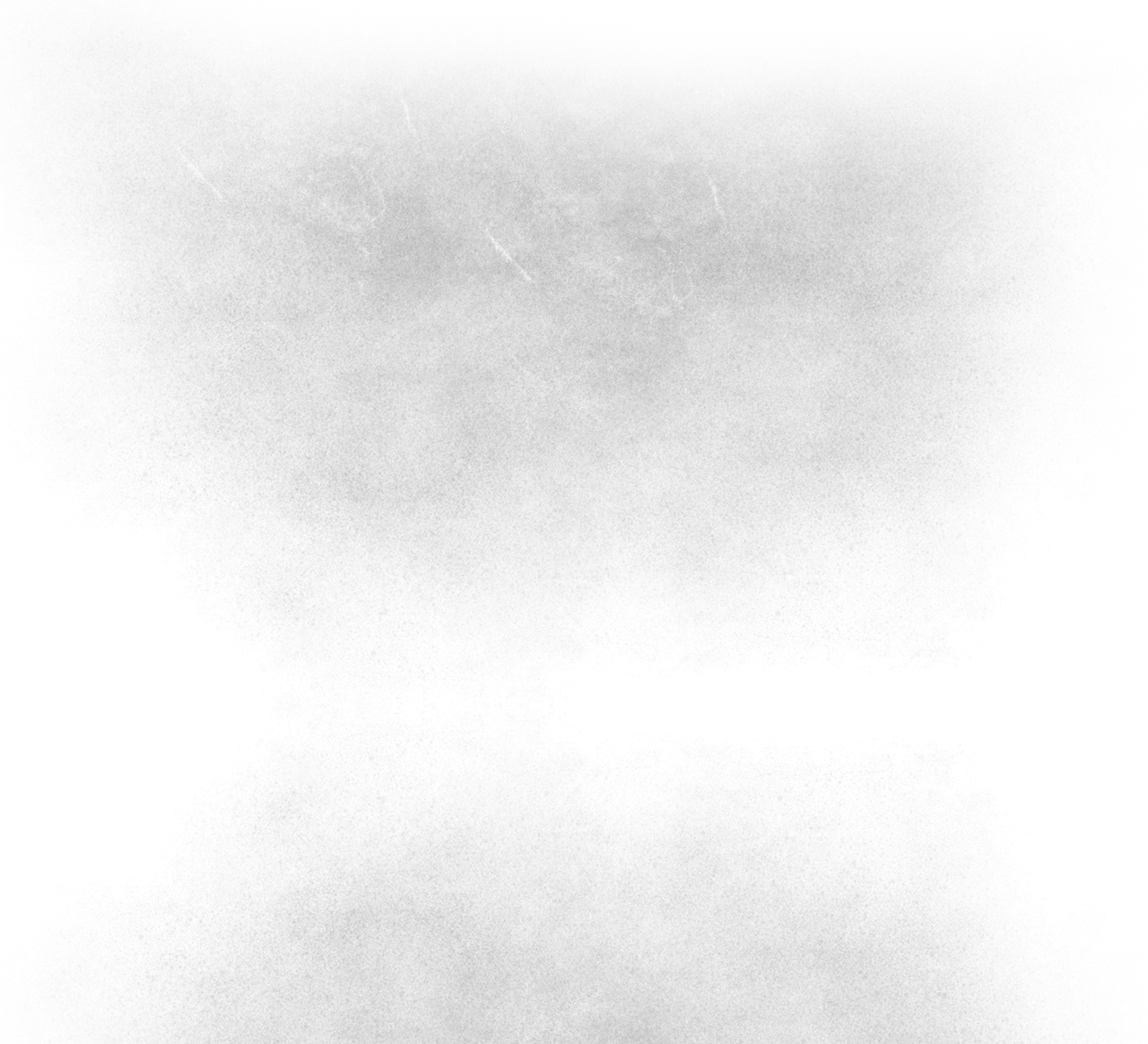

​Turbo C​

We can manipulate the data contained in a variable by using operators.

 Sum = Number1 + Number2; /*add Number1 and Number2, store result in Sum*/
Sum = Number1 - Number2; /*subtract Number2 from Number1, store result in Sum*/
Sum = Number1 / Number2; /*divide Number1 by Number2, store result in Sum*/
Sum = 1 + 2; /*Sum is equal to 3*/
Sum++; /*increment Sum (add one)*/
Sum--; /*decrement Sum (subtract one)*/

We can also copy variables

 Number1 = Number2; /*Number1 now contains same data as Number2*/
​Taking Input

We can use the scanf() function to take input from the person running the program and assign the value to a variable. Here's how it looks:

 scanf("%d", &Number);

 As with printf() we must use the right sign, here we will be dealing with an integer so we use %d. The second parameter is the variable to which we want to assign the value.

 #include <stdio.h>
int main()
{
int Number1, Number2, Sum;
printf("Enter a number:");
scanf("%d", &Number1);
printf("Enter another number:");
scanf("%d", &Number2);
Sum = Number1 + Number2;
printf("The sum of %d and %d is %d", Number1, Number2, Sum);
}

 Now this program is more interesting. It asks the program user for input. It then assigns the first integer value to Number1:
 scanf("%d", &Number1);

 Then it asks them for another number and assigns that to Number2:
 scanf("%d", &Number2);

 The it adds the two values together and prints out the result:

 Sum = Number1 + Number2;
printf("The sum of %d and %d is %d", Number1, Number2, Sum);
But you ask, why does the variable name have a '&' in front of it? Well when we're dealing with scanf() we must preceed the variable name with that '&' sign. You must do this because you are storing the input directly into a memory location. For now, just don't forget the '&'.
​
Let's do it with a char.

 #include <stdio.h>
int main()
{
char Letter;
printf("Enter a letter:");
scanf("%c", &Letter);
printf("Your letter is %c", Letter);
}

scanf()is a very useful function, play around with it for a while, try modifying the program a little, maybe take two letters. When you're happy continue.
Pointers

Pointers are an important part of C. If you don't understand how pointers work, you use alot of the power and flexibility of C. Pointers are difficult to understand if you don't try.

We define a pointer like so:

int *p;

The * shows that this isn't a variable, it's a pointer. A pointer does what it says, it points. Also, the pointer must be a certain type, as with variables. if it's going to point to an integer, we define it as an integer pointer. Let's have another look:

int num, *p;
num = 10;
p = #

Remember the '&' sign from the last chapter? What this sign means is, instead of assigning the "value" of num to the pointer, we actually assign the address in memory where the data for num is stored. Let's say that again, p will contain the address in memory of num.

Now one thing about pointers is that, logically, they contain two values.

-  p is the location holding the address in memory of num.
- *p is the location pointed to by that address.
​
So if we use p we can manipulate WHERE in memory the pointer is pointing to. Yet if we use *p we can manipulate the data contained within the address that the pointer is poining to. If we alter *p, we also alter the variable it points to, let's look at an example. p contains the pointed-to address, *p is another name for the variable it is pointing to. Get it? I hope so, it's kind of confusing at first.

#include <stdio.h>
int main()
{
int num, *p; /* define variable and pointer */
num = 7; /* number now equal to 7 */
p = # /* p contains ADDRESS IN MEMORY of num */
*p = 5; /* num now equals 5 */
num = 3; /* num AND *p now equal 3 */
}

 Think of *p as an alias for the variable it points to, it allows you to manipulate the data within that variable. While p controls which variable, which address in memory we are pointing to. Pointers allow us to jump around memory addresses and manipulate the data in them, allowing us to get into a lower-level, into the workings of things. To fully understand we should discuss memory addresses.
Operations